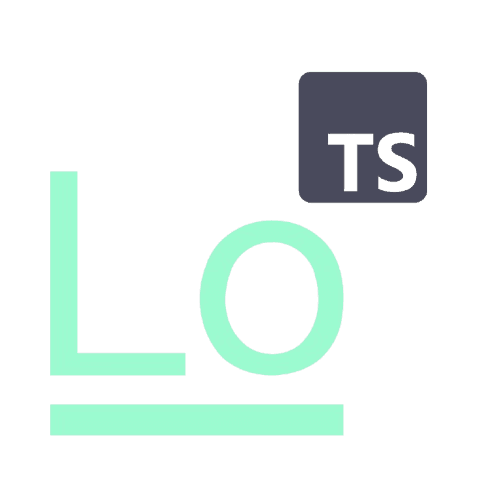
Mastering Lodash Debounce in TypeScript
Learn how to use Lodash debounce in TypeScript to optimize input handling and API calls.
Author
Waseem Issa
Date
19.Nov 2024
Read
6 Min
When building modern web applications, user input is often at the heart of many interactions. Whether it's searching for a product, filtering data, or typing in a chat box, each keystroke can trigger an action. In cases where users are typing in search fields, sending an API call after every single keystroke can be both inefficient and frustrating.
This is where debouncing comes into play—a technique that optimizes how functions are invoked in response to rapid user input. By using Lodash's debounce function in TypeScript, you can significantly improve performance, reduce unnecessary API calls, and provide a smoother, more responsive user experience.
What is Debouncing and Why is it Important?
Debouncing is a programming technique used to limit the rate at which a function is executed. It ensures that a function is only called once after a specified delay has passed since the last invocation. This is particularly useful in scenarios like search fields, where you don't want to trigger an API call for every character typed but instead wait until the user has stopped typing.
In the context of search functionality, debouncing helps to:
- Prevent excessive API calls: When typing quickly, without debouncing, each keystroke could trigger a separate API call, resulting in performance issues.
- Improve user experience: By reducing unnecessary loading or delays, the app feels more responsive and fluid.
- Optimize server load: Reduces the number of calls made to the server, preventing overloading your backend with requests.
How Lodash Debounce Improves Search API Calls
Lodash is a powerful utility library that simplifies many common tasks in JavaScript and TypeScript. One of its most useful methods for optimizing user input handling is debounce
. This function delays the invocation of the provided function until a specified time has passed since the last invocation, thus minimizing the number of times an expensive API request is fired.
Why Wait for the User to Stop Typing?
Waiting for the user to finish typing before making the API call is crucial for several reasons:
- Efficiency: If an API call is made on every keystroke, the server will handle far more requests than necessary, creating unnecessary load. This can slow down both the user experience and backend performance.
- Improved User Experience: Imagine typing a query and seeing the page refresh or loading indicators for each letter you type. It would be distracting and slow. By debouncing the API call, users experience a more responsive interface.
- Minimize Overhead: Continuously calling an API with each keystroke causes multiple redundant requests, increasing the load on the server and wasting resources. With debounce, only the final query (after the user has stopped typing) triggers the request.
Implementing Debounce in a React Component
Here’s how you can use Lodash’s debounce
function within a React component to handle search input efficiently:
import React, { useState } from 'react';
import _ from 'lodash';
const SearchComponent: React.FC = () => {
const [searchTerm, setSearchTerm] = useState('');
// Debounced search function
const debouncedSearch = _.debounce((query: string) => {
// API call can be made here
console.log('API call with:', query);
}, 500); // Delay of 500ms
const handleSearchChange = (event: React.ChangeEvent) => {
const value = event.target.value;
setSearchTerm(value);
debouncedSearch(value); // Call the debounced function
};
return (
Your component goes here
);
};
export default SearchComponent;
In this example:
- As the user types in the input field, the
handleSearchChange
function is triggered. - The actual search logic (
debouncedSearch
) is only executed after the user has paused typing for 500ms, preventing unnecessary API calls during the typing process.
Advantages of Debouncing in Search Inputs
By implementing debounce in your search functionality, you gain the following benefits:
- Reduced Server Load: Instead of firing an API request for every keystroke, requests are batched together, reducing the number of calls to the backend.
- Faster Application: By limiting how often the API is called, the application feels faster and more responsive.
- Improved User Experience: Users are more likely to stay engaged with the search feature because they won’t experience lag or interruptions from continuous loading states.
Conclusion
Debouncing is an essential optimization technique, especially for user input fields like search boxes. By using Lodash debounce in TypeScript, you can prevent unnecessary API calls, improve the performance of your app, and create a smoother, more intuitive user experience.
When dealing with search functionality or other rapidly changing input, it’s crucial to allow some time for the user to finish typing before sending a request. This way, you ensure that your application remains efficient, responsive, and scalable.